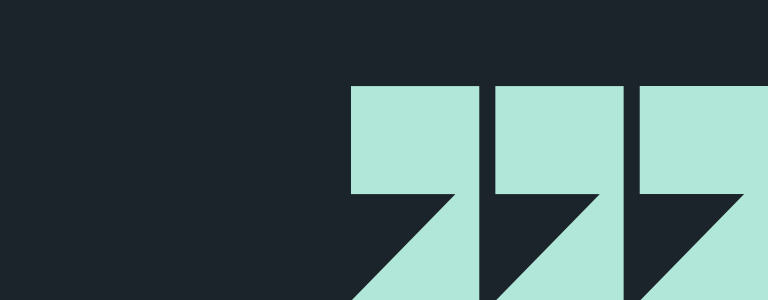
Blog
Insights across the world of tech including software engineering, digital product strategy and development, data and AI, and emerging technologies.
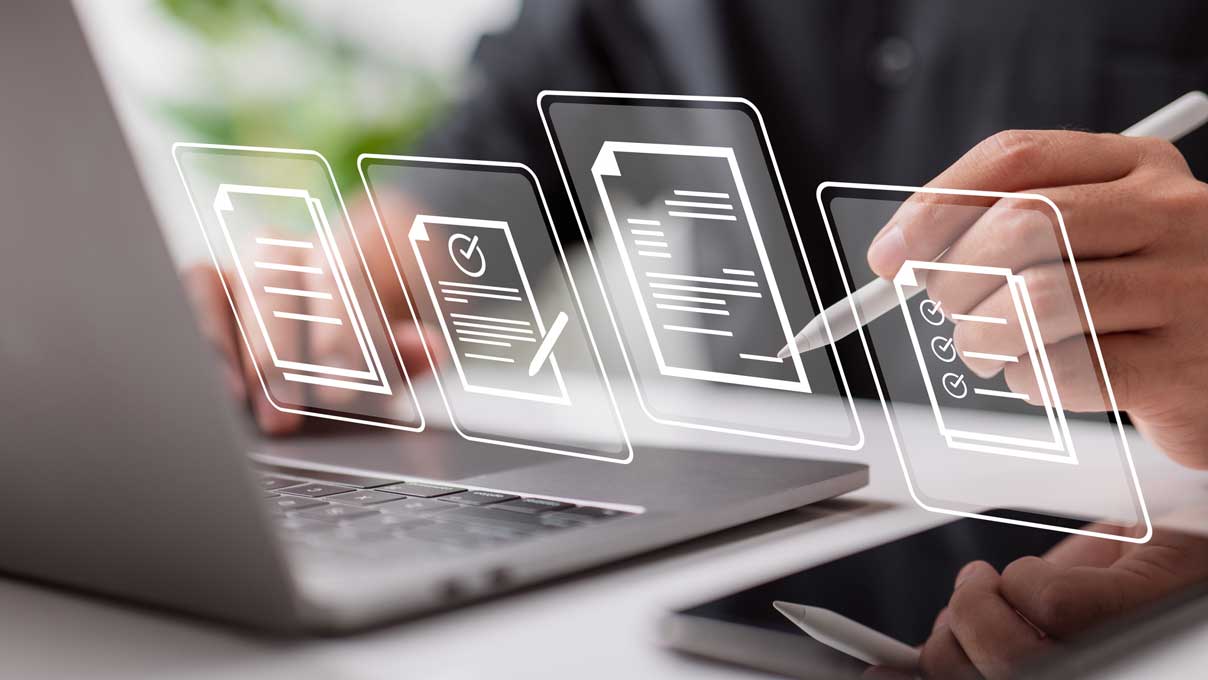
MVP/PoC
The Upside of Feature Creep: How to Manage It Effectively
September 4, 2024It’s time to see feature creep in a whole new light. Feature creep doesn’t have to be a bad thing. It can be to the benefit of your MVP engagement if managed correctly.
Read more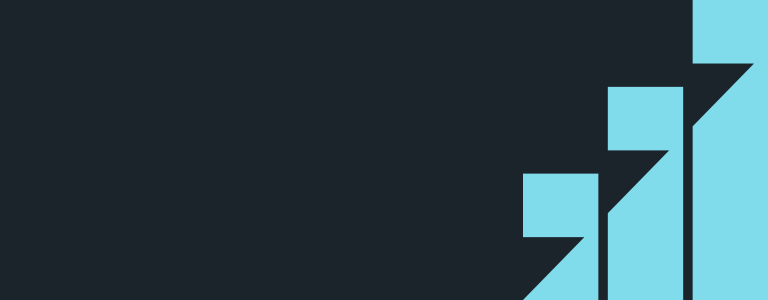
Stay in Touch
Keep your competitive edge – subscribe to our newsletter for updates on emerging software engineering, data and AI, and cloud technology trends.