Using the Protractor Automation Tool to Test AngularJS Applications
One of the applications that we are working on is being built using pure AngularJS. AngularJS is a framework that is gaining in popularity because it extends the functionality of HTML and can be used to quick launch applications. In our quest for a functional automation framework to automate the UI and functionality of the web application, we zeroed in on the Protractor Automation tool.
Protractor, formally known as E2E testing framework, is an open source functional automation framework designed specifically for AngularJS web applications. It was introduced during AngularJS 1.2 as a replacement of the existing E2E testing framework. The Protractor automation tool is also recommended by AngularJS for scenario testing. To read more about AngularJS, please refer to their “Guide to AngularJS Documentation.”
Salient features of the Protractor Automation tool:
- Built on the top of WebdriverJS and Selenium server
- Introduced new simple syntax to write tests
- Allows running tests targeting remote addresses
- Can take advantage of Selenium grid to run multiple browsers at once
- Can use Jasmine or Mocha to write test suites
Protractor is a wrapper (built on the top) around Selenium WebDriver, so it contains every feature that is available in the Selenium WebDriver. Additionally, Protractor provides some new locator strategies and functions which are very helpful to automate the AngularJS application. Examples include things like: waitForAngular, By.binding, By.repeater, By.textarea, By.model, WebElement.all, WebElement.evaluate, etc.
Protractor Installation
For this project, the Protractor framework is being used and configured on a Windows environment. Below are the steps for installation:
Install Protractor Globally
- Step 1: Download and install NodeJS. http://nodejs.org/download/. After installation make sure its path is configured correctly, so that command execution can find Node.
- Step 2: Open the command prompt and type in the following command to install protractor globally.
npm install –g protractor
Install Protractor Locally
You can install protractor locally in your project directory. Go to your project directory and type in the following command in the command prompt:
npm install protractor
Verify Installation
To verify your installation, please type in the command
Protractor --version
If Protractor is installed successfully then the system will display the installed version. Otherwise you will have to recheck the installation.
- Step 3: If you don’t have the Selenium server on your machine, then download the latest version onto your machine. If you want to run tests on different browsers, then please download the respective drivers as well. For example, Chrome driver will come in handy to run tests on the Chrome browser.
Write your test cases:
Create a new folder and name it “test cases” or anything as per your convenience.
· Create a test case spec file. For example: “grid_spec.js” file below, you can create multiple spec files as per your automation scope.
grid_spec.js
describe('HealthSense POC Grid', function() { beforeEach(function() { browser.get('http://Your Application URL'); ptor = protractor.getInstance(); }); it('should click on the grid link', function() { element(by.xpath('//a[contains(text(),"Grid")]')).click(); expect(element(by.xpath('//h1')).getText()).toEqual('Master Grid'); }); it('should enter ID in filter', function() { ptor.actions().sendKeys(protractor.Key.HOME).perform(); element(by.model('Model.ID')).sendKeys('10'); results = element.all(by.repeater('value in testValues')); expect(results.count()).toEqual(5); element(by.model('Model.ID')).clear(); ptor.actions().sendKeys(protractor.Key.SPACE).perform(); }); it('should change the number of records per page to 10', function(){ element(by.xpath('//select')).click(); element(by.css('option[value="10"]')).click(); results1 = element.all(by.repeater('value in testValues')); expect(results1.count()).toEqual(10); }); });
· Create a configuration: The configuration file is used to define some parameters which will be passed to Protractor to execute our spec files. Please note:
- Selenium server details (required)
- Location of our spec files (required)
- Browser capabilities for spec files (required)
- Jasmine node configuration options (required)
- Jasmine reporter configuration options (optional)
Below is a sample configuration file:
Conf.js
exports.config = { //The address of a running selenium server. seleniumAddress: 'http://localhost:4444/wd/hub', //Capabilities to be passed to the webdriver instance. capabilities: { 'browserName': 'chrome' }, //Specify the name of the specs files. specs: ['grid_spec.js'], //Options to be passed to Jasmine-node. jasmineNodeOpts: { onComplete: null, isVerbose: false, showColors: true, includeStackTrace: true } };
Execute your tests:
Once you are ready with your test cases and configuration file, the next step is to execute the test and see the results. Please follow these steps to execute the tests:
Run selenium server
- Make sure the Selenium server (which is specified in the configuration file) is running.
If you want to run the Selenium server manually, then run the following command
java -jar selenium/selenium-server-standalone-2.35.0.jar -Dwebdriver.chrome.driver=./selenium/chromedriver
Run tests
Finally, you are ready to run the tests. Type the following command in the command prompt:
protractor Conf.js
- You should be able to see the test execution progress and its status on the command line and also generate the test reports in an XML format. Please see the next section for details.
Generate Reports:
Protractor supports integration with Jasmine reporters. Jasmine reporters have a collection of JavaScript Jasmine reporter classes. We have used the JUnitXMLReporter which provided the test execution results in XML format. Please follow the following steps to configure the reporter.
Install Jasmine reporter
Install Jasmine reporter by typing the following command on command promptnpm install -g jasmine-reporters
Update Configuration file
After successful installation, create a report output directory inside your project folder and specify the report output directory name in the configuration file. Add the following code into your configuration
file.require('jasmine-reporters'); jasmine.getEnv().addReporter(new jasmine.JUnitXmlReporter('outputdir/', true, true));
XML output report
After execution of your tests again, you can find the test execution report XML in the output directory. Below is a sample of an XML report.
Recent blog posts
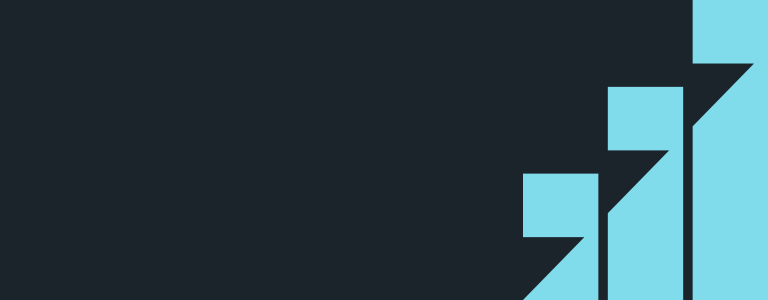
Stay in Touch
Keep your competitive edge – subscribe to our newsletter for updates on emerging software engineering, data and AI, and cloud technology trends.